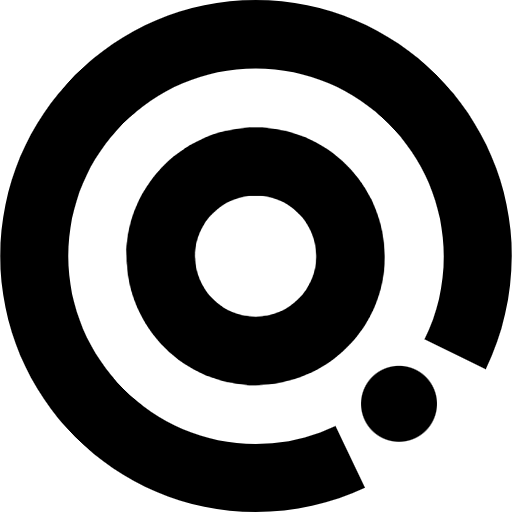
Managing Content
Rest API
Rest API
The Moxly API allows querying users and databases for suppliers who have agreed to be distributed.
Since the API is based on REST principles, it's very easy to write and test applications. You can use your browser to access URLs, and you can use pretty much any HTTP client in any programming language to interact with the API. Features
- REST API
- Supports JSON for requests and responses
- Simple data model
- Secured by SSL and API Key
APIs are designed for programmers, so if you don't speak any computer language, we have solutions that should be easier for you to use.
Specification format
This specification is built upon OpenAPI Specification version 3.0.x.
The queries provided use sample data. For your REST requests you need to obtain secret keys for authorization.
Security
All requests must use HTTPS, the API is not available on non-SSL/TLS ports.
All API calls require a valid <SECRET_TOKEN> Key. It must be passed as a HTTP Bearer authorization, with all requests, using the following format:
curl --location 'https://<YOUR_SITE>/rest-api/test' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
If you are a user of the Saas platform, then you need to substitute the URL of your site as <YOUR_SITE>.
How to get a SECRET TOKEN
Open your application Settings and select Rest API settings in left sub menu.
This query will return information about the application:
curl --location 'https://<YOUR_SITE>/rest-api/application' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
Return:
{
"id": xxx,
"unique_string_id": "xxxxxxxx",
"name": "Test Subscription",
"description": "Test Subscription",
"pwa": 1,
"ios": 1,
"android": 1,
"bundle_id": "com.xxxxxx.xxxxxxx.g5ctiggv5d",
"size": 0,
"version": "1.0.0",
"screen_mode": "both",
"admob_enabled": 0,
"admob_banner_id": null,
"admob_interstitial_id": null,
"reward_video_ad": null,
"background_image": null,
"background_image_mode": "repeat",
"background_image_size": "auto",
"application_css": null,
"one_signal_id": null,
"one_signal_api_key": null,
"one_signal_enabled": 0,
"mixpanel_token": null,
"mixpanel_enabled": 0,
"google_services_json": "",
"google_services_plist": "",
"use_crashlytics": 0,
"icon_background_color": "#FFFFFF",
"splashscreen_background_color": "#FFFFFF",
"splashscreen_show_spinner": 1,
"splashscreen_spinner_color": "#FFFFFF",
"splashscreen_timeout": 0,
"blocked": 0,
"disabled": 0,
"need_first_build": 0,
"need_www_build": 1,
"request_www_build": 0,
"build_now": 0,
"use_default_privacy": 1,
"privacy_text": "",
"default_language": "en",
"google_analytics_view_id": null,
"last_export_time": null,
"created_at": "2023-10-28T10:36:32.000000Z",
"updated_at": "2023-11-12T20:56:10.000000Z",
"google_map_key_ios": null,
"google_map_key_android": null,
"google_map_key_web": null,
"transitions": null,
"transition": null,
"external_domain": null,
"external_domain_use": 0,
"ionic_design": 2,
"start_page_next_page": 7332,
"start_page_next_page_unreg": 7332,
"status_bar_hide_ios": 0,
"status_bar_hide_android": 0,
"status_bar_transparent": 0,
"auth_mode": "wordpress"
}
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/application',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/application", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Creating, editing and deleting application users.
Get all application users:
curl --location 'https://<YOUR_SITE>/rest-api/users?limit=3&page=1&sort_field=name&sort_order=desc' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
Response:
[
{
"id": 661,
"name": "username1",
"phone": "",
"balance": 0,
"role": 1,
"avatar": "https://secure.gravatar.com/avatar/26c57938c0a88b6bf0c8bb00a09ac0c9?s=96&d=mm&r=g",
"blocked": 0,
"last_date": null,
"created_at": "2023-11-05T17:35:22.000000Z",
"updated_at": "2023-11-05T17:35:22.000000Z",
"lastname": "",
"custom_fields": {
"username": "username1",
"nickname": "username1",
"locale": "ru_RU",
"description": "",
"url": ""
},
"email": "email@test.com1",
"push_id": null,
"session": null,
"wordpress_id": null,
"hook_insert_active": 1,
"hook_update_active": 1,
"hook_delete_active": 1,
"wp_token": null,
"wp_user_id": 7
},
{
"id": 664,
"name": "Name21",
"phone": "",
"balance": 0,
"role": 1,
"avatar": null,
"blocked": 0,
"last_date": null,
"created_at": "2023-11-23T18:48:33.000000Z",
"updated_at": "2023-11-24T06:54:10.000000Z",
"lastname": "Last Name21",
"custom_fields": {
"custom_fields": {
"ddduser_name": "Username test123"
}
},
"email": null,
"push_id": null,
"session": null,
"wordpress_id": null,
"hook_insert_active": 1,
"hook_update_active": 1,
"hook_delete_active": 1,
"wp_token": null,
"wp_user_id": 0
},
{
"id": 674,
"name": "Name2",
"phone": "",
"balance": 0,
"role": 1,
"avatar": null,
"blocked": 0,
"last_date": null,
"created_at": "2023-11-24T06:56:23.000000Z",
"updated_at": "2023-11-24T06:56:23.000000Z",
"lastname": "Last Name2",
"custom_fields": {
"ddduser_name": "Username test123"
},
"email": null,
"push_id": null,
"session": null,
"wordpress_id": null,
"hook_insert_active": 1,
"hook_update_active": 1,
"hook_delete_active": 1,
"wp_token": null,
"wp_user_id": 0
}
]
This request can use parameters:
- limit - Limit, default 10
- page - Page number, default 1
- sort_field - Sorting field (not required)
- sort_order - Sort direction (asc or desc, not required)
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/users?limit=3&page=1&sort_field=name&sort_order=desc',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var requestOptions = {
method: 'GET',
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/users?limit=3&page=1&sort_field=name&sort_order=desc", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Get a specific user by his ID:
curl --location 'http://<YOUR_SITE>/rest-api/users/<USER_ID>' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
Response:
{
"id": 663,
"app_id": 1380,
"name": "Alex1",
"mail": "test1@test.com",
"phone": "",
"balance": 0,
"role": 1,
"avatar": "https://secure.gravatar.com/avatar/94fba03762323f286d7c3ca9e001c541?s=96&d=mm&r=g",
"blocked": 0,
"last_date": null,
"created_at": "2023-11-08T20:13:45.000000Z",
"updated_at": "2023-11-08T21:37:43.000000Z",
"lastname": "Govorkov1",
"custom_fields": {
"username": "mutest",
"nickname": "mutest",
"locale": "en_US",
"description": "",
"url": ""
},
"email": "test1@test.com",
"push_id": null,
"session": null,
"wordpress_id": null,
"hook_insert_active": 1,
"hook_update_active": 1,
"hook_delete_active": 1,
"wp_token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpc3MiOiJodHRwczovL3dwLm53Y29pbi5pbyIsImlhdCI6MTY5OTQ3OTQ2MywibmJmIjoxNjk5NDc5NDYzLCJleHAiOjE3MDAwODQyNjMsImRhdGEiOnsidXNlciI6eyJpZCI6IjkifX19.bLmd-8Kzx3CKsPIUBPTm_WUiKEeA1sAehUW2xO8XEo4",
"wp_user_id": 9
}
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'http://<YOUR_SITE>/rest-api/users/663',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'follow'
};
fetch("http://<YOUR_SITE>/rest-api/users/663", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
To create a new user you need to send a POST request:
curl --location 'https://<YOUR_SITE>/rest-api/users' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer <SECRET_TOKEN>' \
--data '{
"name":"Name2",
"lastname":"Last Name2",
"custom_fields": {
"ddduser_name":"Username test123"
}
}'
You must pass filled in custom fields as parameters. If some fields are not sent in the request, they will remain empty.
If the request contains a password field, the user will be set to the passed password, otherwise the user will be assigned a random password.
A successful request will return the resulting user record, otherwise an error will be returned with a status other than 200.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/users',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"name":"Name2",
"lastname":"Last Name2",
"custom_fields": {
"ddduser_name":"Username test123"
}
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json',
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var raw = JSON.stringify({
"name": "Name2",
"lastname": "Last Name2",
"custom_fields": {
"ddduser_name": "Username test123"
}
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/users", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
PUT Request to edit user. In the address bar you must pass the user ID whose data you want to change:
curl --location --request PUT 'https://<YOUR_SITE>/rest-api/users/<USER_ID>' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer <SECRET_TOKEN>' \
--data '{
"name":"Name21",
"lastname":"Last Name21",
"custom_fields": {
"ddduser_name":"Username test123"
}
}'
You must pass filled in custom fields as parameters. Only those fields that are in the request will be updated.
If the request contains a password field, the user will be set to the passed password, otherwise the user will be left with the old password.
A successful request will return the resulting user record, otherwise an error will be returned with a status other than 200.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/users/664',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS =>'{
"name":"Name21",
"lastname":"Last Name21",
"custom_fields": {
"ddduser_name":"Username test123"
}
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json',
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var raw = JSON.stringify({
"name": "Name21",
"lastname": "Last Name21",
"custom_fields": {
"ddduser_name": "Username test123"
}
});
var requestOptions = {
method: 'PUT',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/users/664", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
To delete a user, you must send a DELETE request with the user ID in the URL:
curl --location --request DELETE 'https://<YOUR_SITE>/rest-api/users/<USER_ID>' \
--header 'Authorization: Bearer <SECRET_TOKEN>' \
--data ''
If the deletion is successful, a response with status 200 will be returned, otherwise an error will be returned with status 4ХХ.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/users/662',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'DELETE',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var raw = "";
var requestOptions = {
method: 'DELETE',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/users/662", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Requests for working with embedded Moxly databases.
Each request must contain a unique database secret key.
The Secret Access Key can be obtained in its Database settings, in the Api and Hooks tab:
The key cannot be changed, but it can be recreated.
Be careful when regenerating the key - after this all your connections with the old key will stop working.
Get database contents:
curl --location 'https://<YOUR_SITE>/rest-api/database?limit=2&page=1&sort_field=Name&sort_order=asc&secret=%3CDATABASE_SECRET_KEY%3E' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
The request may contain the following parameters:
- secret - Database secret key (required)
- limit - Limit, default 10
- page - Page number, default 1
- sort_field - Sorting field (not required)
- sort_order Sort direction (asc or desc, not required)
This request return list of items:
[
{
"id": 125003,
"categories": [
124995,
124997
],
"Name": null,
"Thumbnail_icon": null,
"Main_Image": null,
"Ingredients": null,
"Directions": null,
"Recipe_History": null
},
{
"id": 124998,
"categories": null,
"Name": "Classic burger",
"Thumbnail_icon": [
"https://app.moxly.io/storage/application/5522-0ifg4xiyfd/resources/dH9lA7T29r.png"
],
"Main_Image": [
"https://app.moxly.io/storage/application/5522-0ifg4xiyfd/resources///dH9lA7T29r.png",
null,
null
],
"Ingredients": {
"en": "<p>Here is a list of ingredients typically used to make a classic burger:</p>\n\n<ol>\n\t<li>Ground Beef (or another type of meat or vegetarian alternative)</li>\n\t<li>Salt</li>\n\t<li>Black Pepper</li>\n\t<li>Burger Buns</li>\n\t<li>Lettuce</li>\n\t<li>Tomato</li>\n\t<li>Onion (optional)</li>\n\t<li>Cheese (optional)</li>\n\t<li>Pickles (optional)</li>\n\t<li>Condiments such as Ketchup, Mustard, and/or Mayonnaise</li>\n</ol>\n\n<p>Feel free to customize your burger with other toppings or ingredients to match your personal taste preferences!</p>"
},
"Directions": {
"en": "<p>Here's a simplified way to prepare a classic burger:</p>\n\n<ol>\n\t<li>\n\t<p><strong>Prepare the Meat</strong>:</p>\n\n\t<ul>\n\t\t<li>In a bowl, mix 1 pound of ground beef with salt and black pepper to taste. Optionally, you can add minced garlic or onion for extra flavor.</li>\n\t\t<li>Divide the meat into 4 equal portions and shape each portion into a patty.</li>\n\t</ul>\n\t</li>\n\t<li>\n\t<p><strong>Cook the Patties</strong>:</p>\n\n\t<ul>\n\t\t<li>Preheat a grill or stovetop griddle over medium-high heat.</li>\n\t\t<li>Place the patties on the grill or griddle and cook for about 4-5 minutes on each side for a medium-done burger, or to your desired level of doneness.</li>\n\t</ul>\n\t</li>\n\t<li>\n\t<p><strong>Prepare the Buns and Toppings</strong>:</p>\n\n\t<ul>\n\t\t<li>While the patties are cooking, slice the burger buns in half and prepare your desired toppings such as lettuce, tomato, onion, pickles, and cheese.</li>\n\t</ul>\n\t</li>\n\t<li>\n\t<p><strong>Assemble the Burgers</strong>:</p>\n\n\t<ul>\n\t\t<li>Toast the buns on the grill or in a toaster until they are lightly browned.</li>\n\t\t<li>Place a patty on the bottom half of each bun.</li>\n\t\t<li>Add your choice of toppings and condiments such as ketchup, mustard, or mayonnaise.</li>\n\t\t<li>Place the other half of the bun on top.</li>\n\t</ul>\n\t</li>\n\t<li>\n\t<p><strong>Serve</strong>:</p>\n\n\t<ul>\n\t\t<li>Serve immediately while hot, with sides like fries or a salad.</li>\n\t</ul>\n\t</li>\n</ol>\n\n<p>This is a basic recipe, and there's a lot of room for creativity. You can experiment with different types of meat, cheeses, toppings, and condiments to find what you enjoy the most!</p>"
},
"Recipe_History": {
"en": "<p>The evolution of the burger recipe has deep historical roots, traversing cultures and continents over centuries. Here's a concise history:</p>\n\n<p><strong>Early Precursors:</strong></p>\n\n<p>In Ancient Rome, around 1 AD, a dish resembling the burger called 'Isicia Omentata' was known, where a beef patty was mixed with ingredients like pine kernels and wine1.<br />\nIn Germany, by at least the 17th century, a dish known as "Frikadelle" existed, which was akin to a hamburger.</p>\n\n<p><br />\n<strong>19th Century Developments:</strong></p>\n\n<p>In the early 19th century, many European emigrants to the US were introduced to minced beef in a manner reminiscent of their home cuisines, particularly from the Hamburg region, hence leading to dishes like Hamburg-style American fillet3.<br />\nBy the late 19th century, Hamburg steak, a patty of chopped beef, became popular in many New York restaurants. The dish was sometimes served raw with onions and bread crumbs, reflecting the steak tartare tradition.</p>\n\n<p><br />\nIn 1834, a dish referred to as "Hamburg steak" was listed on the menu at Delmonico's Restaurant in New York, indicating its presence in the American culinary scene from early on.</p>"
}
}
]
Or it will return an erroneous status and an error message.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/database?limit=2&page=1&sort_field=Name&sort_order=asc&secret=%3CDATABASE_SECRET_KEY%3E',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/database?limit=2&page=1&sort_field=Name&sort_order=asc&secret=<DATABASE_SECRET_KEY>", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Get a item from the database for a specific ID:
curl --location 'https://<YOUR_SITE>//rest-api/database/<ITEM_ID>?secret=9a7a09b1-74a8-4dc5-a109-5d8a78aca539' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
Pass the ID of the requested item in the URL.
Response:
{
"id": 125003,
"categories": [
124995,
124997
],
"Name": null,
"Thumbnail_icon": null,
"Main_Image": null,
"Ingredients": null,
"Directions": null,
"Recipe_History": null
}
Or it will return an erroneous status and an error message.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>//rest-api/database/<ITEM_ID>?secret=9a7a09b1-74a8-4dc5-a109-5d8a78aca539',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>//rest-api/database/<ITEM_ID>?secret=9a7a09b1-74a8-4dc5-a109-5d8a78aca539", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Add a new entry to the database:
curl --location 'https://<YOUR_SITE>/rest-api/database?secret=DATABASE_SECRET_KEY' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer <SECRET_TOKEN>' \
--data '{
"Name":"test testov",
"categories": [
124995,
124997
],
"Recipe_History":"bla bla bla"
}'
The created record will contain only those fields that are in the transmitted request.
Attention, category binding is not checked; you must do it yourself before requesting.
If the operation is successful, the created record or an error message with an erroneous status will be returned.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/database?secret=DATABASE_SECRET_KEY',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{
"Name":"test testov",
"categories": [
124995,
124997
],
"Recipe_History":"bla bla bla"
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json',
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var raw = JSON.stringify({
"Name": "test testov",
"categories": [
124995,
124997
],
"Recipe_History": "bla bla bla"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/database?secret=DATABASE_SECRET_KEY", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
To update an existing record, you need to pass its identifier in the parameters and pass new values to the request:
curl --location --request PUT 'https://<YOUR_SITE>/rest-api/database/<ITEM_ID>?secret=DATABASE_SECRET_KEY' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer <SECRET_TOKEN>' \
--data '{
"Name":"test testov",
"Recipe_History":"bla bla bla",
"categories": [
124995,
124997
]
}'
Updates only those fields that are in the transmitted request.
Attention, category binding is not checked; you must do it yourself before requesting.
If the operation is successful, the updated record or an error message with an erroneous status will be returned.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/database/<ITEM_ID>?secret=DATABASE_SECRET_KEY',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS =>'{
"Name":"test testov",
"Recipe_History":"bla bla bla",
"categories": [
124995,
124997
]
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json',
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var raw = JSON.stringify({
"Name": "test testov",
"Recipe_History": "bla bla bla",
"categories": [
124995,
124997
]
});
var requestOptions = {
method: 'PUT',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/database/<ITEM_ID>?secret=DATABASE_SECRET_KEY", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
To delete a item, you must send a DELETE request with the user ID in the URL:
curl --location --request DELETE 'https://<YOUR_SITE>/rest-api/database/124999?secret=DATABASE_SECRET_KEY' \
--header 'Authorization: Bearer <SECRET_TOKEN>'
If the deletion is successful, a response with status 200 will be returned, otherwise an error will be returned with status 4ХХ.
Example request in PHP:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://<YOUR_SITE>/rest-api/database/124999?secret=DATABASE_SECRET_KEY',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'DELETE',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer <SECRET_TOKEN>'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
Example request in Javascript (with fetch):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer <SECRET_TOKEN>");
var requestOptions = {
method: 'DELETE',
headers: myHeaders,
redirect: 'follow'
};
fetch("https://<YOUR_SITE>/rest-api/database/124999?secret=DATABASE_SECRET_KEY", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));